Introduction:
Spring is an open source framework created to address the complexity of enterprise application development. Enterprise applications has a tremendous need of wiring objects or implementing different behavior of object at runtime that too without imposing much load on application. One of the main advantages of the Spring framework is its layered architecture design, which allows us to wire the objects as and when necessary with reduced maintenance efforts.
Core Features of Spring
- Lightweight:
1.
Spring provides you different modules and
allows you to use any one based to your requirement. Ideally the spring jar is just 2-3 MB.
2.
If you compare Spring with EJB, then you have to write very less
code and configurations too. The beauty of Spring is that you can actually
focus on business logic whereas in EJB you have to write lot of code along with
business logic which makes the code bulky and tightly coupled.
3.
Through Spring you are playing with POJO which
do not depends on Framework and it improves the testability of your code.
4.
And, Spring provides seamless integration with frameworks, third
party libraries etc.
- Inversion of control (IOC):
The Inversion of Control (IoC) and Dependency Injection (DI)
patterns are all about removing dependencies from your code.. No need to directly connect your components and services
together in program, instead just describe which services are needed by which
components in a configuration file/xml file. The Spring IOC container is then
responsible for binding it all up.
- Aspect oriented (AOP):
Aspect oriented
programming refers to the programming paradigm which isolates
secondary or supporting functions from the main program's business logic. AOP
is a promising technology for separating crosscutting concerns, something
usually hard to do in object-oriented programming. The application's modularity
is increased in that way and its maintenance becomes significantly easier.
- Container:
Spring
contains and manages the life cycle and configuration of application objects.
- MVC Framework:
Spring
comes with MVC web application framework, built on core Spring functionality.
This framework is highly configurable via strategy interfaces, and accommodates
multiple view technologies like JSP, Velocity, Tiles, iText, and POI. But other
frameworks can be easily used instead of Spring MVC Framework.
- Transaction Management:
Spring
framework provides a generic abstraction layer for transaction management. This
allowing the developer to add the pluggable transaction managers, and making it
easy to demarcate transactions without dealing with low-level issues. Spring's
transaction support is not tied to J2EE environments and it can be also used in
container less environments.
- JDBC Exception Handling:
The
JDBC abstraction layer of the Spring offers a meaningful exception hierarchy,
which simplifies the error handling strategy. Integration with Hibernate, JDO,
and iBATIS: Spring provides best Integration services with Hibernate, JDO and
iBATIS
Architecture:
Spring
is well-organized architecture consisting of seven modules. Modules in
the Spring framework are:
- Spring
AOP:
One of the key components
of Spring is the AOP framework. AOP is used in Spring:
- To
provide declarative enterprise services, especially as a replacement for
EJB declarative services. The most important such service is declarative
transaction management, which builds on Spring's transaction
abstraction.
- To
allow users to implement custom aspects, complementing their use of OOP
with AOP
- Spring
ORM:
The ORM package
is related to the database access. It provides integration layers for popular
object-relational mapping APIs, including JDO, Hibernate and iBatis.
- Spring
Web:
The Spring Web module is
part of Spring?s web application development stack, which includes Spring MVC.
· Spring DAO:
The DAO (Data Access Object) support in Spring is primarily
for standardizing the data access work using the technologies like JDBC,
Hibernate or JDO.
· Spring Context:
This package builds on the beans package to add support for
message sources and for the Observer design pattern, and the ability for
application objects to obtain resources using a consistent API.
· Spring Web MVC:
This is the Module which provides the MVC implementations
for the web applications.
· Spring Core:
The Core package
is the most import component of the Spring Framework.
This component provides the Dependency Injection features. The BeanFactory provides a factory pattern which separates the dependencies like initialization, creation and access of the objects from your actual program logic.
This component provides the Dependency Injection features. The BeanFactory provides a factory pattern which separates the dependencies like initialization, creation and access of the objects from your actual program logic.
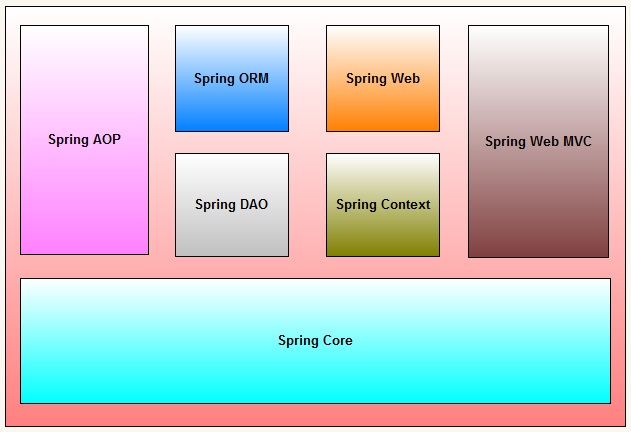
In my next blog post I will let you dive in Dependency injection(ioc) in spring
Keep posting your queries if any